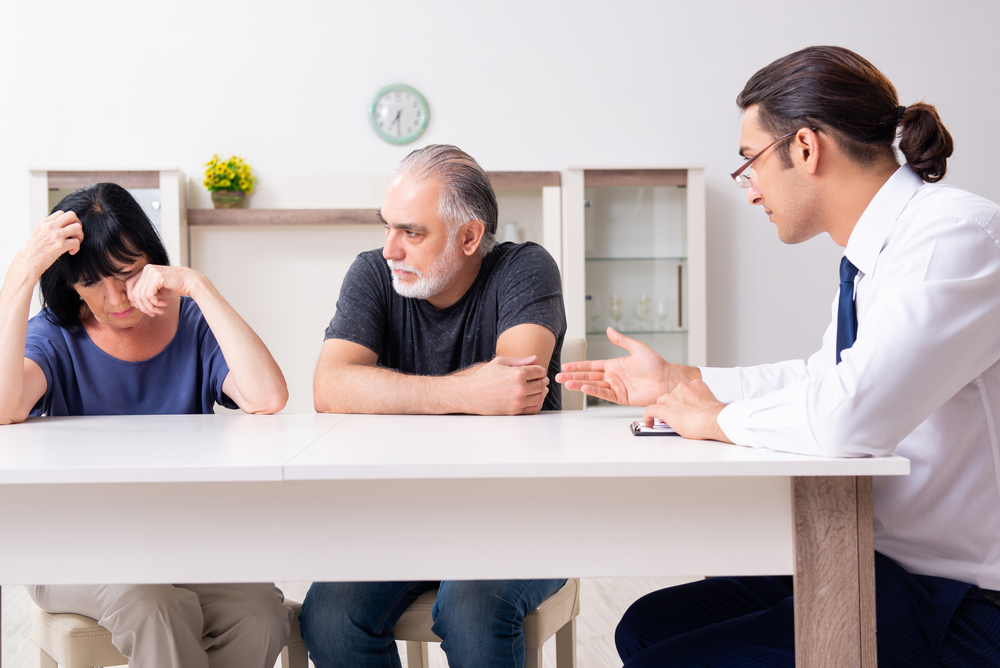
What Are Bankruptcy Exemptions in the State of Michigan?
Bankruptcy is an option for Michigan residents facing overwhelming debt, but many have concerns about losing assets during the process. There are protections for a filer’s property, so what are bankruptcy exemptions in the State of Michigan?
Bankruptcy exemptions in Michigan protect essential assets like your home (up to $40,475 in equity), vehicles (up to $3,725 in equity), and personal property. They ensure you retain critical possessions while discharging debts. Choose federal or state exemptions based on your needs.
Exemptions under federal versus state law may provide a higher level of protection, but the rules are complex. It’s wise to trust a Michigan bankruptcy lawyer for assistance with identifying exemptions and properly applying them when filing your petition. Plus, an overview of the relevant concepts is useful.
What are Exemptions in Michigan Bankruptcy Cases?
Bankruptcy exemptions in Michigan refer to legal protections that allow individuals to retain certain assets during bankruptcy proceedings. These exemptions help prevent debtors from losing everything they own, so they have the essentials to rebuild their financial lives.
Exemptions under the US Bankruptcy Code apply differently based on the type of bankruptcy filed. In Chapter 7 bankruptcy, they determine which assets are protected from liquidation. For Chapter 13 bankruptcy, exemptions influence the repayment plan by dictating the value of assets creditors cannot access.
Understanding exemptions is critical for anyone considering bankruptcy in Michigan. They are designed to provide a financial safety net while balancing the interests of creditors and debtors.
Why are Bankruptcy Exemptions Important in a Chapter 7 Bankruptcy?
In Chapter 7 bankruptcy, exemptions are crucial because they determine which assets can be retained by the filer. This type of bankruptcy involves liquidating non-exempt property to pay creditors, making exemptions a lifeline for maintaining stability.
For example, Michigan exemptions might protect your primary residence, car, and essential household items. Without these protections, individuals could face undue hardship, losing not just luxury items but critical resources necessary for daily living.
Bankruptcy exemptions also streamline the process by reducing disputes over which assets are subject to liquidation. This enables the process to remain fair and efficient for all parties involved.
What are Federal Versus State Exemptions in Michigan?
Michigan offers a unique approach to bankruptcy exemptions by allowing filers to choose between federal and state exemption systems.
- Federal exemptions are uniform across the United States and provide specific asset protection limits.
- State exemptions, however, reflect Michigan’s localized approach to addressing the needs of its residents.
Each system includes a range of protections for personal property, real estate, and other assets. For instance, the federal system may offer higher limits for some categories, while Michigan’s state exemptions may excel in others, like homestead protections. Understanding the differences between these options is key to maximizing the benefits of filing for bankruptcy in Michigan.
Does Michigan Allow Filers To Use Federal Bankruptcy Exemptions?
Yes, Michigan allows bankruptcy filers to choose between federal and state exemption systems. This flexibility gives filers the opportunity to select the option that provides the most advantageous protection for their assets.
For example, if a filer owns significant equity in their home, Michigan’s homestead exemption might offer better protection. Alternatively, federal exemptions might benefit someone with diverse personal property holdings.
However, filers must stick to one system entirely—they cannot mix and match exemptions from both. Consulting with a bankruptcy attorney helps you make the right choice based on your financial situation.
How Do I Use Exemptions When Filing for Bankruptcy in Michigan?
Using exemptions effectively during bankruptcy in Michigan requires careful planning and accurate documentation. Filers must identify all assets and determine which are protected under their chosen exemption system.
Exemptions are claimed on specific bankruptcy forms submitted to the court. Errors in this process can lead to disputes or loss of legal protections, so attention to detail is critical. It’s critical to comply with Michigan bankruptcy laws, but you must also secure the maximum benefit from available exemptions. This helps individuals preserve vital assets and avoid unnecessary complications.
What are Key Exemptions Available Under Michigan Law?
Michigan law provides several critical exemptions to help protect assets during bankruptcy. For instance:
- Homestead Exemption: This allows individuals to shield their primary residence, offering significant protection for homeowners.
- Personal Property Exemptions: These rules cover essentials like household goods, clothing, and tools of the trade.
- Motor Vehicle Exemption: Michigan also provides an exemption for cars, light trucks, and other passenger vehicles.
- Retirement Accounts: Certain individual retirement accounts and pensions are fully exempt, promoting long-term financial security. Plus, in Michigan, retirement benefits paid to a recipient are generally protected.
These exemptions are designed to safeguard basic needs, making it possible for individuals to recover financially after bankruptcy in Michigan.
How Does the Michigan Homestead Exemption Work?
The Michigan homestead exemption allows individuals to protect equity in their primary residence during bankruptcy. This exemption applies to homes, condos, and even some mobile homes, provided the property is the filer’s primary residence.
Michigan law permits up to $40,475 in equity protection for most individuals, with higher limits available for married couples filing jointly or those with disabilities. This safeguard means filers won’t lose their homes due to bankruptcy proceedings.
The homestead exemption provides a critical foundation for financial recovery by preserving stable housing, a key element of rebuilding after bankruptcy.
Can I Use a Motor Vehicle Exemption in Michigan?
Michigan’s motor vehicle exemption allows bankruptcy filers to protect up to $3,725 of equity in a personal vehicle. This is particularly valuable for individuals who rely on their cars for commuting, work, or essential errands.
If the equity in your car exceeds the exemption limit, options may exist to protect the vehicle, such as reaffirming the loan or working out payment arrangements. Filing under Chapter 13 bankruptcy can also help retain vehicles with higher equity. This exemption supports the mobility and independence needs of filers throughout the bankruptcy process.
What are Michigan’s Personal Property Bankruptcy Exemptions?
Michigan law provides exemptions for essential personal property, enabling filers to keep necessary items and allowing for basic living needs during bankruptcy. Assets that you can protect, up to a reasonable value, include:
- Household goods
- Furniture
- Appliances
- Clothing, some jewelry, and personal belongings
- Tools of the trade, such as those required for employment
Michigan’s personal property exemptions aim to minimize the disruption to daily life caused by bankruptcy while providing creditors with equitable treatment. These provisions help create a balance between protecting filers and addressing their financial obligations.
What Happens to Property You Can’t Exempt in a Michigan Bankruptcy?
Property that cannot be exempted in a Michigan bankruptcy may be subject to liquidation under Chapter 7. The bankruptcy trustee oversees the sale of such non-exempt assets to repay creditors.
However, certain arrangements can help mitigate loss. For example, Chapter 13 bankruptcy allows debtors to retain non-exempt property while repaying creditors through a court-approved repayment plan. Understanding what property is at risk and planning accordingly is essential to minimize losses and secure the best possible outcome during bankruptcy proceedings.
How Do I Protect Financed Property in Chapter 7 Bankruptcy?
Protecting financed property, such as a car or furniture purchased on credit, during Chapter 7 bankruptcy involves reaffirmation agreements or redemption.
- Reaffirmation agreements allow filers to keep financed property by agreeing to continue payments under the original loan terms.
- Redemption involves paying the creditor a lump sum equal to the property’s current fair market value, which may be less than the amount owed.
Both options require court approval for purposes of fairness. However, these strategies help filers retain essential assets while resolving their financial difficulties.
Consult with an Attorney About Michigan Bankruptcy Exemptions
It’s critical to know the basics of bankruptcy exemptions in Michigan, but there are many additional details that could impact your case. At Kostopoulos Bankruptcy Law, our experienced attorneys focus on guiding clients through the complexities of bankruptcy law. Our goal is to protect your rights while also maximizing assets under Michigan’s exemption rules.
Call us today at 877-969-7482 for a free no-obligation consultation. We’re prepared to help you find the best path forward toward financial freedom.
Related Content:
- How Do You File for Bankruptcy in Michigan?
- Debunking Bankruptcy Myths in Michigan
- How Long Does it Take to File Bankruptcy in Michigan?
- How Much Does Chapter 7 Bankruptcy Cost in Michigan?
FAQs About Bankruptcy Exemptions in Michigan
These protections allow individuals to retain vital assets needed for daily living and future financial recovery. The specific exemptions depend on the chosen exemption system: federal or state.
However, if you choose to use federal bankruptcy exemptions instead of Michigan’s state exemptions, the federal system does include a wildcard exemption of up to $1,475 in any property, plus up to $13,950 of unused homestead exemption if they don’t use it all for their home.
These protections permit individuals to retain access to essential income sources, enabling them to meet daily living expenses during and after bankruptcy. The specifics of these exemptions may vary depending on the exemption system chosen.